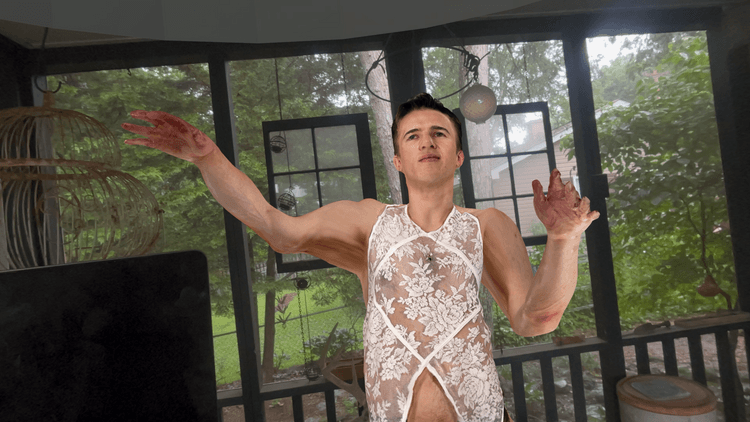
Tips on Tasks from ChatGPT 4o
Improving a real-time frame rate by asking ChatGPT 4o to optimize your code.
I used ChatGPT to improve some of the code I was using to communicate from an iPad to a visionPro over multipeer connectivity.
Here are some of the suggestions I used:
Subscribing to the update loop to update the transforms:
subscription = content.subscribe(to: SceneEvents.Update.self, on: nil, componentType: nil) { event in
if browserModel.nextJointData != browserModel.firstJointData {
updateEntities()
}
}
Using a detached background task to decode the data:
func performDecodeAsyncOperation() async -> [String:[JointData]]? {
if let data = lastData as Data? {
return await Task.detached(priority: .background) { [weak self] in
return self?.createDecodeTask(with: data)
}.value
}
return nil
}
Cancelling the decode task correctly:
// Optionally, a method to start the task
func startDecodeTask() async throws {
cancelCurrentDecodeTask()
decodeTask = Task {
if Task.isCancelled {
print("returning cancelled task")
return nil
}
return await performDecodeAsyncOperation()
}
try await handleDecodeTaskResult()
Task { @MainActor in
decodeTask = nil
}
}
// A method to handle the task result
func handleDecodeTaskResult() async throws {
if Task.isCancelled {
print("Returning cancelled task with no value")
decodeTask = nil
return
}
let value = await decodeTask?.result.map { result in
switch result {
case .some(let data):
return data
case .none:
print("Task failed with error")
return [String:[JointData]]()
}
}
if let jointData = try value?.get(), let decodeTask = decodeTask, !decodeTask.isCancelled {
Task { @MainActor in
if nextJointData != jointData {
firstJointData = nextJointData
nextJointData = jointData
print("Swapped joint data")
}
}
}
}
public func cancelCurrentDecodeTask() {
if let decodeTask = decodeTask, !decodeTask.isCancelled {
cancelDecodeTask()
print("cancelled decode task")
}
decodeTask = nil
}
// Optionally, a method to cancel the task
func cancelDecodeTask() {
decodeTask?.cancel()
}
Compressing and decompressing my messages: do { let jsonData = try JSONSerialization.data(withJSONObject: rawData)
// Compress the data
// Send data with user-initiated priority
let compressedData = try (jsonData as NSData).compressed(using: .lz4)
try multipeerSession.send(compressedData as Data, toPeers: multipeerSession.connectedPeers, with: .unreliable)
} catch {
print(error)
}
Task {
do {
lastData = try (data as NSData).decompressed(using: .lz4)
print("Did receive data \(data) at \(displayLinkTimestamp)")
} catch {
print(error)
}
}
Using a .userInitiated thread for sending messages from the iPad:
sendTask = Task(priority: .userInitiated) {
if Task.isCancelled {
print("Canceled task")
return
}
model.send(rawData:model.jointRawData)
print("Sent model")
}
Copyright 2024, Secret Atomics LLC